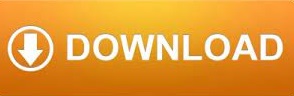

In PHP this might look like this: // composer require predis/predis We do this because this is being used in a distributed context (we have many workers performing API calls), and we don't want to write when we already exceeded our limits. Also note that, in the same transaction as reading the list of timestamps, we add a new timestamp to the list (the ZADD command). It serves as the key for our Redis sorted set. Instead of using the actual OAuth access tokens (and duplicating them to Redis), you might want to use an identifier or hash of the token instead as $accessToken. EXEC will execute all previously queued commands and restore the connection state to normal.EXPIRE $accessToken $slidingWindow to reset the expiry date for this sorted set of timestamps (for the current OAuth Token).ZADD $accessToken $now $now to add a log for the current API call that we're about to do.ZRANGE $accessToken 0 -1 to get a list of all API call timestamps that happened during the window.ZREMRANGEBYSCORE $accessToken 0 ($now - $slidingWindow) to remove API call timestamps that were done before the start of the window.
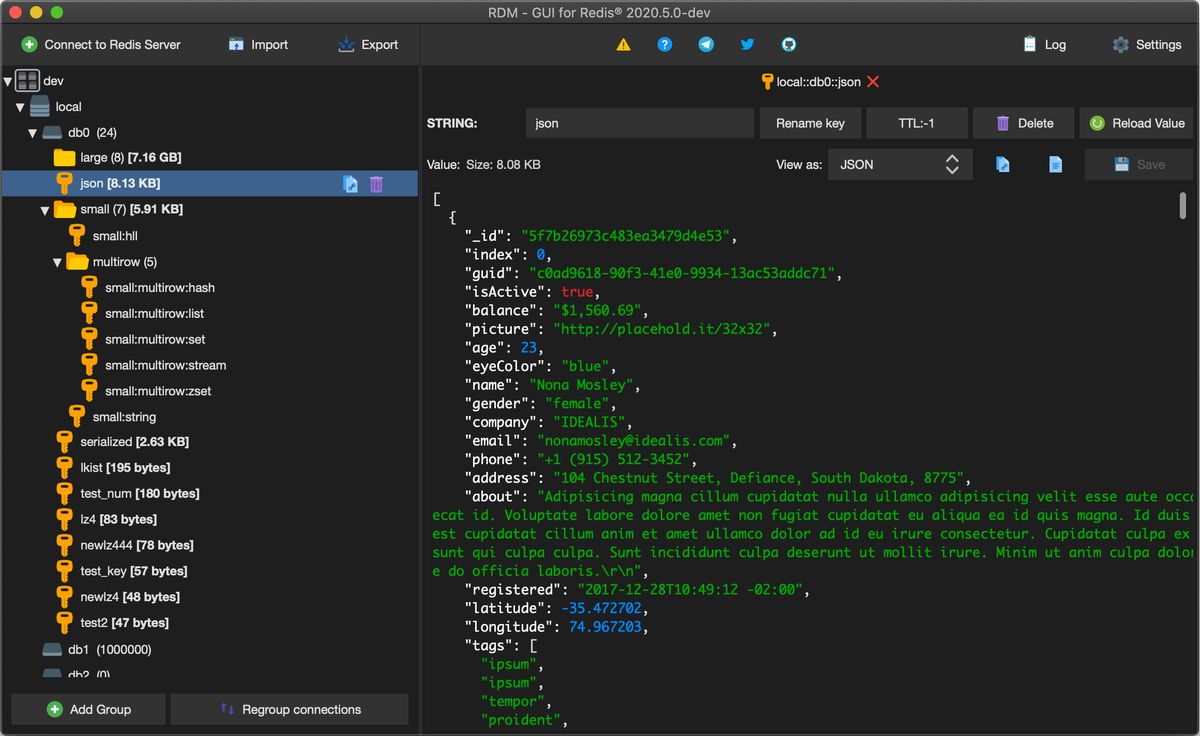
Subsequent commands will be queued for atomic execution using EXEC.
#REDIS WINDOWS CLIENT INSTALL#
If you're on a mac, you can just $ brew install redis to get it. Assuming you already have Redis installed, start a server: $ redis-server.
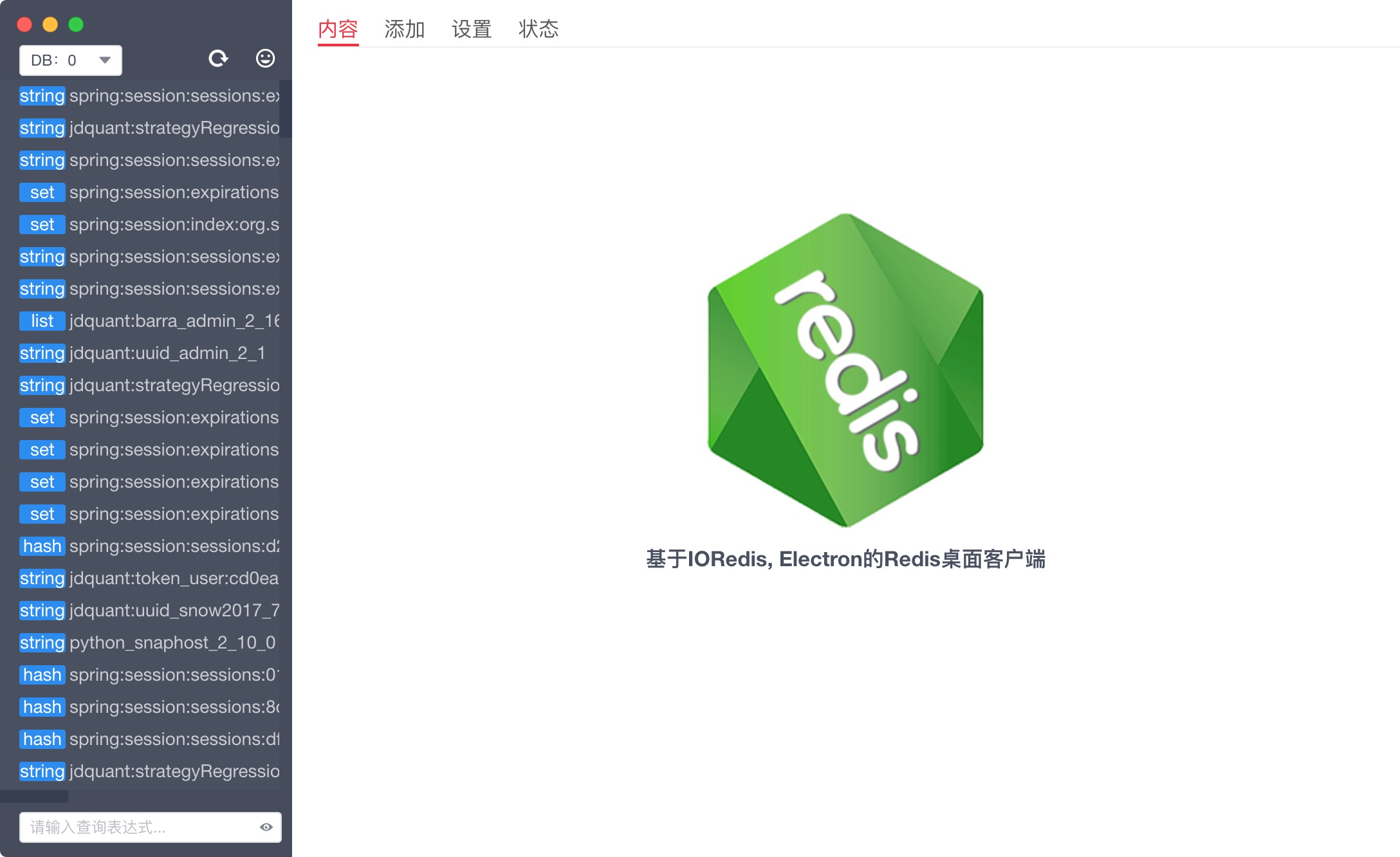
We're ready to translate our algorithm to Redis commands. Redis is a key/value store that supports lists, sets, sorted sets and more. What about Redis? Yes, I like where this is going. Sadly Memcached doesn't have the concept of an array or list (we could serialize an array using our favourite programming language). What about Memcached? Yes, that's also an option.

Maybe we could use MySQL or PostgreSQL? Yes, we could but then we would need a system that periodically removes outdated timestamps since neither MySQL or PostgreSQL allow us to set a time to life on a row. Now we need some kind of database to store a list of timestamps grouped per access token. Let's say we did 4413 API calls in the last hour then we're allowed to do 587 more at this moment.
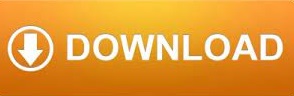